Bitly’s V4 API – Sample PHP Code
Recently Bitly have rolled out version 4 of their API, and if you’re using the old API, it is quite a change.
While users were asked to move from older versions to the new version before March 1st 2020, the version 3 API was to hang around until March 31st 2020 to give people a little extra time to move.
Given the current COVID-19 situation, this discontinuation has been put on hold for the time being as per an email sent out to API users.
Nevertheless, it is absolutely time to move to the new version to ensure that you can still automate the shortening of your URLs.
Given my professional working life, I’ve worked with very similar API implementations, so for me it wasn’t a big deal to change over.
Here is the simple function I wrote for PHP, to do a basic URL shorten. Your PHP installation will need to have cURL and JSON libraries installed and enabled.
function shorten_url ($access_token,$group_guid,$shorten_domain,$shorten_url) {
# setup the JSON payload
$json_payload = @json_encode(Array(
"group_guid"=>"".$group_guid."",
"domain"=>"".$shorten_domain."",
"long_url"=>"".$shorten_url.""
));
# initialise cURL handle
$curl_handle = @curl_init();
# define cURL parameters
@curl_setopt_array($curl_handle,Array(
CURLOPT_URL => "https://api-ssl.bitly.com/v4/shorten",
CURLOPT_RETURNTRANSFER => TRUE,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => TRUE,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => $json_payload,
CURLOPT_HTTPHEADER => Array (
"Host: api-ssl.bitly.com",
"Authorization: Bearer ".$access_token."",
"Content-Type: application/json",
),
));
# execute the cURL request
$json_output = @curl_exec($curl_handle);
# decode the output and get the HTTP response code
$json_decoded = @json_decode($json_output);
$http_code = "".@curl_getinfo($curl_handle,CURLINFO_HTTP_CODE)."";
# return results for further processing
return(Array("json"=>$json_decoded,"http"=>$http_code));
}
The inputs are relatively straight forward:
$access_token – this is the same as your existing OAuth application access token – this has not changed, at least in my case.
$group_guid – this is the only new piece of information you might need. While you can query for this value via the API, for most people the simplest way to get it is to open into your Bitly account in a web browser, where you will find the GUID in the URL, as per this image:
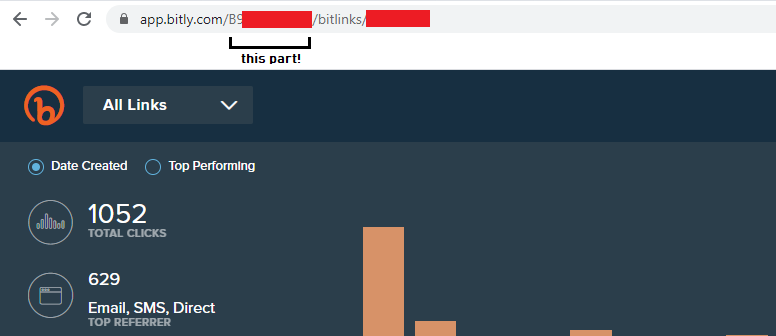
$shorten_domain – if you have a custom domain – (for example, I have the domain “mwyr.es” for shortening purposes) – this is the value you need here. If you don’t have a custom domain, just use “bit.ly”.
$shorten_url – this is the URL you wish to shorten – simple!
So now you can call the function, with the required information.
Firstly, the input data required by the API is converted to JSON via the json_encode() function.
Secondly, we set up the cURL handle, and then supply it with the required data. Note the JSON data is passed within the “CURLOPT_POSTFIELDS” variable, and that the access token is passed as the “Authorization: Bearer” header in the “CURLOPT_HTTPHEADER” variable.
Then we execute with curl_exec() – and store and return the results for further processing.
That’s it!
To understand the returned JSON – (including the generated short link) – and the HTTP response codes, refer to the excellent Bitly API v4 documentation.